Javascript
Javascript Background
Javascript is what provides functionality and interactivity to the website. With only HTML and CSS, we are limited to making static webpages. As the name implies, static webpages don't change. They just display information. The only time they can change is if the developer manually makes the changes in the markup or style sheets. But once we add JS, we can serve dynamic webpages. We can write apps that run in our browser and allow our webpages to do alot more than serve up fancy images and text. Javascript allows us to change the webcontent on the fly.
Client-side vs Server-side Programming
Client-side programming means that you let the client's browser do all the heavy work. You send the HTML, CSS, and JS to their browser and the browser processes the script into HTML and renders it onto the viewport. The downside to this method is that different browsers may process the script a little differently, meaning your webpage may deliver a different experience to different browsers. Server-side programming eliminates that by letting the web server process the script into HTML first before sending the web page to the client. The client receives only HTML/CSS code so all users get the same experience. You can even combine client-side and server-side programming, commonly used in form data validation. The client-side script handles whether each form entry is being entered correctly and completely while the server-side handles the processing of the data. While normally a client-side language, with the introduction of node.js, JS works as a server-side programming language too.
Building your own web server
For actual production sites, you should use a dedicated web hosting service. But for personal development, you can set up your own server environment. One of the most popular packages is XAMPP.
Adding Javascript to your Webpage
There are two main ways to add JS to your webpages: inline and externally.
Inline Javascript
Inline javascript means adding the javascript directly to your HTML using the <script>
...</script>
tags; all your javascript just goes between the tags.
While you could place the tags anywhere in your HTML, it's important to know that the location affects when your javascript will run. If we want our JS to run before anything on the page is rendered, place the tags in the head
. If we want it to run after everything is rendered, place the script
tags after the body
. Placing our script somewhere in the body means it will run after everything before it in the body is rendered. Everything after it in the body will not render until the script is done. There's a good chance this is not what you want so to be safe, just stick with putting the script in either the head or after the body. Another thing to note is that if you put your script in the head, make sure that you don't reference any elements in the body of the HTML. This is because when the script runs, the browser has yet to render anything below it (i.e. the document body) so referring to body elements at this point will result in an error. However, there are ways to bypass this by essentially telling the browser to wait until everything is rendered before running the script. One way is to just add the defer
attribute to the script tag in the HTML.
External Javascript
We can write all of our script in a separate .js
file and link to that in the HTML, just like external style sheets.
<script src="javascript.js"></script>
The benefit of an external script is that we can quickly apply it to multiple pages at once, rather than typing the script out in the HTML for every web page. And if we need to change the script later, we can just make the changes in that one document instead of going to and making the changes on every web page that uses the script.
Javascript Structuring
In terms of script structure, JS is similar to CSS. There is no containers or tags to set up like HTML; you just start putting down code right away. Here are some key things to note when writing Javascript.
- Javascript is case sensitive. Ex.var1 and Var1 are two different variables
- Use "camelCase" when naming variables using more than one word. The first word is lower case, and each subsequent word is capitalized. Ex. helloWorld. Read more about variables here.
- Constants (a special data type in JS used to store values that don't change) should be named in all-caps.
- Adding semicolons (;) to the end of each code statement is not necessary but is encouraged to help keep your code readable
Thats the end of the basics with JS. Now we'll start looking at what we can do with JS. In the code examples below, custom user input will be put in italics so as to differentiate between them and JS keywords.
Working with Variables and Datatypes
Datatypes
Datatypes are a way to define the type of data we're working with. In JS there are 5 main datatypes:
- Strings
- Numbers
- Boolean
- Null
- Undefined
The 'null' datatype is used to specifically mean 'nothing' or 'empty' and is used to intentionally assign 'no value' to variables. The 'undefined' datatype also means 'empty'; unassigned variables are 'undefined'. The difference between these two is that 'null' is intentionally assigned to variables while the 'undefined' datatype means that the variable doesn't have anything assigned to it at all.
The core of programming is getting data and doing something with it and then displaying it back to the user. To work with data, we need some way to capture and store it so that we can work on it later. This is where variables come in. Think of variables as personally-labelled containers. We can use variables to store our data and whenever we want to use that data, we just call the variable by its name. Note: information stored in JS variables only exist as long as the user has the web page loaded. The momment the user exits the web page, that data is lost forever. To store data long-term, we'll need to transport that data into a database like an SQL server.
JS Variables
var varName;
- You declare variables with the
var
keyword. There are some limitations on how your variables can be named:- Variables can only contain letters, numbers, _ , and $
- Variables can only begin with a letter, _ , or $.
- Variables are case sensitive.
varName = value;
- You assign values to variables using the
=
sign. In JS, this is known as the assignment operator. There are different datatypes you can assign to a variable:- Numerical values, which can be assigned as is
- String values, which must be enclosed in "" or ''. You can also set a modular string using `` (the symbol part of the tilde key). Modular strings allow you to embed variables in them like so:
`My favourite colour is: ${favColour}`
. The variable's value will then be incorporated in the string value. - Arrays of values, which must be enclosed in [] and each value must be separated by a comma. Arrays are a biggie so more on them later.
null
andundefined
values
var varName = value;
With ECMAScript, we can also declare variables using const
for constants and let
for block-scope variables. Constant variables can't be changed after assignment. Normally variables are function-scoped or gobal depending on where they are declared. However with let
, you can have the same variable name hold different values just by being in different code blocks.
String methods
stringVar.startsWith("prefix")
- Returns a boolean on whether the string starts with the specified prefix. Case sensitive.
stringVar.endsWith("suffix")
- Returns a boolean on whether the string ends with the specified suffix. Case sensitive.
stringVar.includes("substring")
- Returns a boolean on whether the specified substring exists within the string. Case senstiive.
stringVar.search("substring")
- Searches for the specified substring in the string and returns the index of its first occurrence.
stringVar.repeat(#)
- Repeats the string the specified amount of times.
Arrays
Arrays are unique in that they can store multiple data values (called elements) under a single variable. There are some important characteristics when working with arrays
- Array elements do not have to be all the same datatype.
- Array elements are indexed starting at 0 for the first item and so on
- You refer to specific values in the area by referencing their index number, like so:
favourite_word=dictionary[46];
- You can assign new values to array elements by referencing their index number:
dictionary[4]="apple";
Array Methods
arrayName.reverse()
- Reverses the positions of the items in the array
arrayName.shift()
- Reverses the first item in the array
arrayName.unshift(item1,item2...)
- Adds new items to the front of the array.
arrayName.pop()
- Removes the last item from the array.
arrayName.push(item1,item2...)
- Adds new items to the end of the array.
arrayName.splice(pos#,#toRemove)
- Removes items from a specific part in the array. Two arguments are required. The first is the position number of where you want to start removing elements, non-inclusive. For example, if you want to remove the 5th item from the array, you'd start at position 4. Note that position number is different from index number: positions start at 1, not 0. The second argument is the number of items you want to remove.
arrayName.slice()
- Creates and returns a copy of the array.
arrayName.indexOf(item,index#)
- Returns the index number of the item, if it exists in the array
arrayName.join()
- Returns a string containing all the element values in the array, separated by commas. If you don't want commas, you can write a different separator in the brackets; the method will use whatever you write as the separator instead.
arrayName.forEach()
- Iterates through an array and does whatever function is in the () on each element
arrayName.map()
- Iterates through and performs a function you specify in the () to each element. The changed elements are then placed in a new array which is returned. The array element's value is automatically set as the function argument.
arrayName.includes(value)
- Returns a boolean on whether or not the the specified value is contained in the array
...arrayName
- The spread operator separates out the elements of an array and uses them as arguments in the current context. Ex. you could use this to take the elements of one array and copy them into another array.
let kids = ["Michael", "Mary", "Jennifer"]; let class = ["John", "Calvin", "Priscilla", "Catherine", ...kids];
Destructuring Arrays
Arrays are nice for holding a bunch of information but once all that data is in the array, it can be hard to retrieve specific values. That's where destructuring comes in. Essentially, we break up the array and assign variable names to the elements inside so that we can refer to each of them individually.
var [var1, var2,...] = [element1, element2,...]
Sets
Sets are similar to arrays in that they hold a collection of values that can be any type, in indices starting from 0. The difference between sets and arrays is that elements in a set must be unique. If you try to add an item that's already in the set, nothing will happen.
let books = new Set();
books.add("Sherlock Holmes");
has
method.
var x =books.has("Sherlock Holmes");
You can remove set values by using the delete
method
books.delete("Sherlock Holmes");
Operators
There are three main types of operators: Math operators, Boolean operators and Comparison Operators.
Math Operators
+
- Addition. This is the only Math operator that can also be used with String values; it concatenates two separate strings into one. If one of the arguments is a string, then the other will be treated as a string (regardless of its original type) for concatentation.
-
- Subtraction
*
- Multiplication
/
- Divsion
%
- Modulus. A special division operator that returns only the remainder.
++
- Increment by 1. Note that the time this operation occurs depends on where you put the ++. If you put it in front, then 1 gets added to the value before returning the value. If you put it after (ex. a++), then you return the original value, but add 1 to the value in the background.
--
- Decrease by 1
These other values only perform math related functions. As long as one of the variables is a number, the others can be strings as each of the strings only contains a number.
In cases when your "math" expression is written as a String variable, just use the eval()
method. Plug in the String variable as a paramter and the String will then be evaluated as if it were a math expression.
Boolean Operators
&&
- Logical AND
||
- Logical OR
!
- Logical NOT
Comparison Operators
>
- Greater than
<
- Less than
>=
- Greater than or equal to
<=
- Less than or equal to
==
- Checks if two sides have same value
===
- Checks if two sides have samee value AND same data type
Methods Toolkit
Here are some methods that I've come across that do some pretty useful things.
alert(Message to appear on popup)
- This method generates a popup on the screen, which can be useful for telling your user about something important. They get annoying quick so use them sparingly. If the user has adblockers, there's also a chance they get blocked too.
console.log(Message to show on console)
- This method takes whatever's in the brackets and displays it to the console. Your average user won't be inspecting the console so this method is more for us. It's really helpful for tracking variable values and logic flow at key areas of your program; a great debugging tool. Make sure to remove or comment it out once you deploy!
prompt(Message)
- This method generates a popup that has a text input field. Whatever the user enters can be stored in a variable and manipulated.
eval(Some string)
- This method is useful for evaluating strings as math statements. This can be useful if you're receiving data from a user as a string and you want to process that data as a calculation.
//some comment
- Not really a method but useful regardless. Use
//
to add single-line comments to your code. /*Comment block*/
- If you need to write alot of comments in one place, use the comment block. Everything that goes in between the
/*
and*/
becomes a comment. typeof variableName
- If you ever need to know what type of data a variable is holding, use the
typeof
operator, which returns the data type of the variable varName.setInterval(function1, #miliseconds)
- Assigns an interval to a variable and runs the specified function at every specified time interval in miliseconds.
clearInterval(varName)
- Stops any interval assigned to the specified variable, if it is running.
fetch(URL)
- Fetches from the specified URL, usually used as an API call to fetch some data. This is an asynchronous function call so it is usually linked with
.then()
to process the data whenever it decides to arrive.
Directing Program Flow
If Statements
If
statements let us provide branches in our script; if conditions are met, do this. We can combine them with else
statements to get our program to do
something if conditions are not met. We can chain multiple conditions together with else if
statements.
if(condition){
do something
}
else if (another condition){
do something else
}
else{
do this if nothing else matches
}
Ternary Operator
There's a special operator called a ternary operator that combines variable assignment with an if-else
statement. It looks like ?
.
varName = (condition) ? value1:value2
In a single line, a condition and two values are provided. If the condition is met, the variable is assigned value1. Otherwise, it is assigned value2.
Switches
Logically, switches function pretty much the same as if statements; they provide branches for our code to do different things under different conditions.
switch(expression){
case match1:
do this
break;
case match2:
do this instead
break;
.
.
.
default:
do this if nothing else matches
}
First, the expression's value is calculated. This value is then compared with each of the cases one by one, in descending order. If the case's value (shown as match1, match2 etc) is equal to the expression's value, then that case block gets run. The break
statements are used to skip over the other case blocks once the switch has found a matching one. If no match is found, then the default block is run.
Loops
Loops repeat the same block of code over and over. Once a certain condition is met, like when a variable reaches a certain value, the loop ends. It's critical that at least one of the variables involved in the loop is changing with each iteration. Otherwise, you create an endless loop which does nothing other than stall the program indefinitely and use up resources. There are a few ways to create loops in JS.
do-while Loop
This loop performs the loop once, then checks a condition at the end to see if it should be repeated. Note that regardless of whether the condition is met, the statements in the loop are performed at least once.
do{
code block
}while (condition)
while Loop
This loop checks the condition first before executing the code, so it is possible that the loop doesn't even run once.
while(condition){
code block
}
for Loop
This loop use three values. The first is the setup of a variable that the loop will use to determine its iterations. The second is the condition that that variable must be in, in order for the loop to continue. The third is how that variable is changed at the end of each iteration.
for(varName=value; condition; changes to variable)
{
code block
}
for-in Loop
This loop is a special loop, used to handle iterating through an array. We don't really need to worry about an infinte loop because the loop will only iterate as many times as there are elements in the array. All you need to do is provide a variable name to store the current index value.
for(varName in arrayName)
{
code block
}
All loops repeat their contained code blocks until the specified condition is met. However, it'd be nice if we could stop the loop whenever we wanted. That's where break
and continue
come in. The break
keyword allows us to immediately end the loop, even if the specified loop condition has not been met. This is useful if the loops intention is to find something; once its been found, there's no point in looking at the rest of the objects so we use break
to exit the loop. On the other hand, suppose the current iteration we're on doesn't have what we're looking for. Rather than go through the rest of the loop code block, we can use continue
to immediately move to the next iteration of the loop.
try...catch Statements
This special code block allows us to "try" out a block of code. If we "catch" an error during that block, we provide a second block of code that gets run instead.
try{
code block
} catch(err){
code in case of error
} finally{
code that always gets run regardless of try results
}
The "err" parameter is just a variable name we've provided to hold the error object that gets generated when an error occurs. We can use the variable in our secondary block of code; ex. to alert us of the error that occurred. The finally
block is optional; we can use this block to include any related code that should always run regardless if the try
block fails or not.
Functions
Sometimes when we write a program, sometimes there's a part of it that we'd like to reuse. If we write it as a function, then we can use that chunk of code over and over anywhere we want to without having to type the whole thing out
function fname(parameter1, parameter2,...){
code block
return some value;
}
The function name must be unique, at least within the context of its use. The parameters we give to the function are the only values it has access to; functions are meant to be resusable and standalone after all. We can assign default values to these parameters if we want to; default values will be used if the user does not specify values for any of the parameters. The return
statement allows the function to return some sort of value but is completely optional.
Using the function is pretty simple.
var varName=<fName(parameter1...)
Simply write the function name, and then put the required parameter values in brackets. If the function returns a value, we can assign the function call to a variable and the value it returns will automatically be assigned to the variable. If the function doesn't return a variable, we just call it as is, without variable assignment.
For example:
passwordReset(username2);
Anonymous functions
When we write functions, it helps us package together code that performs a specific task. We can then call that package anywhere in the script to repeat that task. Sometimes the function we write will only be used once. In this situation, its better to use an anonymous function. Anonymous functions have no function name and they will only be used once.
var varName=function(parameter1, parameter2,...){
code block
return some value;
}
Arrow Functions
Sometimes even anonymous functions might seem like overkill if all you want your function to do is perform a simple calculation pass it to a variable. In this case, we can use arrow functions. Arrow functions takes a set of parameters, points those parameters to an action or set of actions, and then returns the value of that calculation.
var multiply =(x, y,...)=>x*y;
console.log(multiply(5,6)); //prints out 30
JS Objects
Creating variables is fine if we have just a few pieces of data we want to store and manipulate. What if we have lots of closely related data? It might be better to bundle all those variables up into an object. Objects in JS are basically bundles of properties and values, of which we can access individually or all at once.
To create an object, we create a function and define all the object properties, values, and even methods. Note the use of the this
keyword to refer to the current object. Typically, this would be some future variable that we haven't created yet and thus, we don't know its name so this
comes in handy.
function Student(name,id,courses) {
this.name=name;
this.id=id;
this.courses=courses;
this.addCourse: function(){
return ++student.courses;
}
};
We can now create a variable and assign it to be the object we just created. Then we can access its object properties and functions using .
notation.
var student1=new Student("John Doe",123456,3);
console.log(student1.name); //prints out "John Doe"
console.log(student1.courses); //prints out 3
student.addCourse();
console.log(student1.courses); //prints out 4
Object spreading
Remember the array spread operator (...
) that let you put the contents of one array into another without nesting the whole array? You can do that to create bigger objects from the contents (property-value pairs) of other objects.
Object destructuring
Lets say we have an object with a bunch of key value pairs. We have a function that only uses some of those key-value pairs. We could set our function constructor to take the whole object and then just use . notation to call the key-values that we want from the object. Another way of doing this would be to specify in the constructor the exact keys we plan to use. Then when we pass the object into the function, those specific key-values are retrieved automatically.
var student = {
name: "John Doe",
GPA: 4
}
function welcomeMsg({name}){
return `Welcome to our school, ${name}!`;
}
console.log(welcomeMsg(student));
Maps
Maps are similar to objects in that you're creating an entity with a bunch of key-value pairs associated with it. However, with maps you can both objects and primitve values as the key or the value in a key-value pair.
let student=new Map([
[new Date(), "Date enrolled"],
["Grades", ["A","B", "A"]]
]);
Notice how a map looks like one big array and how each entry is just a smaller, 2-item array.
You can add new entries manually one by one using the set
method.
student.set("name", "John Doe");
To retrieve a key's value, use the get
method.
student.get("Grades");
If we want to iterate through a map, we can use a for-of loop.
for(let x of student){
do something with each key-value
}
We can combine this with the keys()
or values()
function to retrieve specifically the keys or values from each step in the iteration.
for(let x of student.keys()){
do something with each key
}
Classes
Classes are kind of like blueprints for objects. They allow us to create multiple instances of objects very quickly, without having to type everything out all over again.
To create a class, we use the class
keyword followed by the class name.
class student{
}
Next, we need to define the constructor. We'll use the constructor to help us build each new instance of the class
class student{
constuctor(name, gpa){
this.name=name;
this.GPA=gpa;
}
}
Now, we just add any functions that we want each instance of our class to have. Normally, classes will have a getter and a setter (functions that retrieve property values and functions that set property values.
class student{
constuctor(name, gpa){
this.name=name;
this.GPA=gpa;
}
function setGPA(x){
this.GPA=x;
}
}
Class Inheritance
You can create new classes that inherit features from existing ones by using the extends
keyword.
class gradStudent extends student{
constuctor(){
super("Jane Doe", 3.5);
}
}
The super
keyword is used to reference the constructor from the ancestor class
The DOM
In order for JS to get and manipulate things in your HTML or CSS, it needs a way to find them. This is where the DOM comes in. DOM stands for Document Object Model and it is basically a tree structure containing all of the elements and attributes and CSS styles. The browser creates this tree when rendering the page and because JS runs in the browser, it has access to this tree as well.
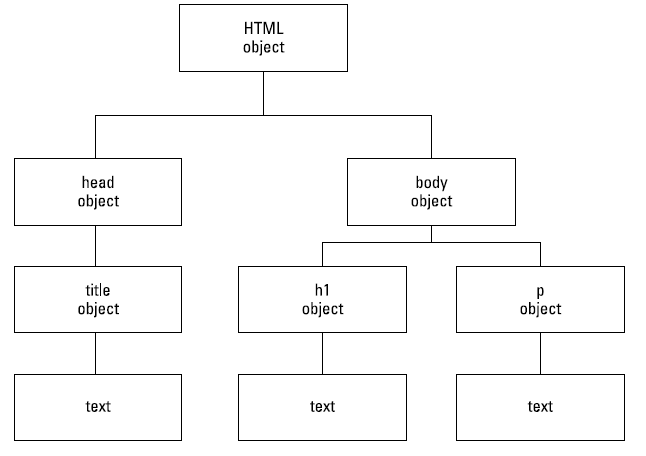
The HTML
element contains all the other elements, so it sits at the root of the DOM. All the other elements get added to the tree in terms of ancestry. Note that text is also considered as a child object in the DOM tree.
Accessing the DOM
In JS, we use the special document
object to access the entire webpage DOM. The document
object has properties we can access and methods we can use in order to add content dynamically to our web page.
document Properties
document.activeElement
- Returns the element that has the focus of the web page
document.anchors
- Returns list of all anchor elements on the web page
document.body
- Gets the body element fo the web page.
document.cookie
- Returns all cookie names and values
document.documentElement
- Returns the HTML element
document.embeds
- Returns a list of all embed elements in the web page.
document.forms
- Returns a list of all form elements in the web page.
document.URL
- Returns the full URL for the web page.
document.title
- Returns the title of the web page.
document.images
- Returns a list of all images in the web page.
document Methods
document.createElement()
- Creates a new element. Note: just sits in memory until you append it to something.
document.createTextNode()
- Creates a DOM text element. Remember, the text part of elements is considered a separate node!
document.getElementbyID(htmlID)
- Retrieves the element object with the specified ID
document.getElementsbyClass(className
- Retrives the list of element objects with the specified class .
document.getElementsByTagname(tagName)
- Retrieves a list of element objects witht the specified element tag
document.querySelector("css selector")
- Gets the first element that matches the specified CSS selector.
document.querySelectorAll("css selector")
- Gets all elements that match the specified CSS selector. Multiple selectors are comma separated.
hasFocus()
- Returns a boolean if the web page is focused in the browser
document.write(text)
- Sends the specified text to the web page for display. Will overwrite all HTML in the section that this is placed.. If you want to change the text content of an element, its better to manipulate that using the object method
innerHTML
. document.writeln(text)
- Sends the specified text to the web page for display, along with a new line character.
Element Methods
element.getAttribute("attribute")
- Returns the element's value for the specified attribute
element.appendChild()
- Adds whatever node is in the brackets as a child to another node.
element.style.styletype
- Accesses the specified CSS style of the element. You can change it by assigning it a string CSS value.
element.value
- Retrieves the value of the selected element. You can change it by assigning it a new value.
element.innerHTML
- Retrieves the content of the selected element. You can change it by assigning it a new value.
These are just an example, there are more you can look up as you need.
DOM Object Methods
Now that we can get the individual objects on the DOM, we can use each object's properties and methods to do things with them. There are a ton of them so I won't bother listing all of them here. Check here for the full list.
Adding DOM Elements in JS
.createElement()
.createTextNode()
Keep in mind that we can chain properties together like a URL to get to the exact thing we want to change:
document.body.style.backgroundColour
Manipulating Form Elements
Text boxes
You can access whatever value is in the text box using its .value
property. For example:
var textbox=document.getElementById("textInput1");
var content = textbox.value;
You can also use the value
property to change the content of the textinput box.
textbox.value="Hello World!";
More text entry related properties can be found here.
Check boxes and Radio Buttons
The checked
property allows you to check if your check box or radio button is checked; it returns a boolean value.
var checkbox_1=document.getElementByID("checkbox1");
var checkbox_status=checkbox_1.checked
You can also manually set whether the checkbox is cheed by assigning it a true or false value.
checkbox_1.checked=true;
Radio buttons are a little different because only one radio button in a group can be checked. Also, because radio buttons in a group all have the same 'name' value, it can be hard to find the exact one you want to mess with. For this, the value
property is important as there will be different values associaed with each radio button.
For more properties and methods, click here.
jQuery: What is it?
When people talk about jQuery, they're just talking about a library of functions. Functions that programmers used time and time again were added to this library to simplify future developemnt. Instead of coding it yourself, just run the associated function from the library!
jQuery: How do I load it?
First you'll need to download the latest production package of jQuery from www.jquery.com. There are four different versions of the package:
- Uncompressed
- Minified
- Slim
- Slim minified
Uncompressed means the full package, uncompressed. Minified means the full package but compressed. Slim removes animation and Ajax functions, but keeps the file uncompressed. Slim minified is basically the slim package but compressed. You'll generally want Minified to keep all the features, while making the file smaller so that your site loads faster
Now that you have jQuery, you'll need to host it on your server so that all your users can use it too when they load your page (T0 BE CONTINUED)
On the other hand, if you don't want to host jQuery you can use a CDN to load the jQuery library from a group of other servers. jQuery hosts its own CDN which you can find here:
code.jquery.com
Google also provides jQuery packages on their CDN: developers.google.com/speed/libraries.
You simply pick your package type and embed the code provided into the start of your script so that it gets loaded before the functions are used
jQuery: How do I write it?
To use jQuery library functions, you'll need to use a special keyword prefix so that the browser knows it has to get that function from jQuery. We can use either jQuery
or a simple $
.
jQuery(jQuery code);
$(jQuery code);
Once the structure is all set up, we just put whatever jQuery function we want inside the ().
jQuery: Finding elements
Finding Elements is easy using jQuery because it uses the same selectors that CSS uses to find elements. The following code picks up the p
element on the webpage.
$("p");
As you can see, we put our selector in "". Here's another example selecting for an ID.
$("#elementID");
jQuery: Replacing data
Once we can select elements, the next step is to change them up in some way.
$("selector").text("Optional text")
- The
.text()
function retrieves the text in the selected element. You can write optional text as a parameter for the.text
function in order to replace the text of the selected element. $("selector").html("HTML code")
- The
.html
function replaces the HTML in the selected element with whatever is written as a parameter. You can pretty much do anything you want HTML wise; rewrite the element to have different values or even replace it with a new element entirely. Note that the parameter must be written as HTML code, which means including the tags and stuff. $("selector").attr("attribute, value");
- The
.attr
function allows you to retrieve and replace the selected element's attributes. If you just want to retrieve the attribute, just omit the value. $("input selector").val("Optional text");
- The
.val
function allows you to retrieve the value attribute for that input. This is extremely handy for validating form data before it gets sent to the server. Putting in an optional text parameter allows you to change the default text for that form input. $("selector").css("property", "value");
- Use the
.css()
function to retrieve and chance CSS style properties for selected elements. The property naming convention is exactly like it is in CSS. If you want to change multiple properties for the same element, you'll need to put them all in {} and seperate each property:value pair with a comma. See the following example:$("p").css({"background-color":"blue", "width":"200px", "color":"white"})
Replacing Text
Replacing HTML
Replacing element attributes
Reading Form Input
Changing CSS
Changing CSS classes
With all these style changes everywhere, in your script and in your CSS, it makes it difficult to change things later. That's why its better to keep JS style changes in the CSS under a custom class name and just change the class of the element when you want to trigger the style change. Here are the jQuery functions we can use:
.addClass("className")
.hasClass("className")
- a boolean.removeClass("className")
.toggleClass("className")
- an on-off toggle
Changing the DOM
Not only can we change existing elements, we can use jQuery to add new elements to the DOM
$("selector").after("HTML code")
- Inserts HTML after the selected element; creates a sibling node.
$("selector").append("HTML code")
- Inserts HTML after the content of the selected element; creates a child node.
$("selector").before("HTML code")
- Inserts HTML before the selected element; creats a sibling node.
$("selector").prepend("HTML code")
- Inserts HTML before the content of the selected element; creates a child node.
$("selector").empty("HTML code")
- Removes all child nodes from the selected node. Note that because text is considered a child node, the element's text is also removed.
$("selector").remove("HTML code")
- Removes the selected node as well as any of its child nodes.
Event-driven Programming
The browser is always keeping an eye and report on what the user is doing in the browser window. We can use this to our advantage and make our site react to whatever the user is doing
Mouse Events in Javascript / HTML5
To detect and trigger events, you can set the following attributes in the HTML elements you want the effect to take place in. A good place to use these is with a generic button
element that places a clickable button on the page.
element.onclick=function
- Primary mouse button has been clicked on the specified element, which triggers the specified function. Note that when specifiying the function, you don't add () at the end.
oncontextmenu
- Secondary mouse button was clicked
onmouseenter
- Mouse pointer has entered a specific area of the window
onmouseleave
- Mouse has left a specific area of the window
onmouseover
- Mouse cursor is hovering over a speciifc object
onmousedown
- Primary mouse button is pressed down
onmouseup
- Primary mouse button has been released
Keyboard events in Javascript/HTML5
onkeydown
- A key is being pressed down
onkeyup
- A key has been released
onkeypress
- A key has been pressed and released
The disadvantage to detecting events in this way is that only one event can be bound to an element at a time. If you want an event to trigger multiple functions, use an event listener.
Page events in HTML
There are even some events that deal with the page itself, like when its printed, when its loaded, or when its viewed offline. These aren't as interactive as the others but you can read more about them here.
Event Listeners
Another way to add event detection is to add an event listener using pure JS. The advantage to this is that we don't have to add any attributes to our HTML elements. The event detection and reaction all happens in the javascript.
To add an event, select the element in Javascript and then use the addEventListener()
method, which has the following structure:
selectedElement.addEventListner("event", function1, function2...);
As you can see, the addEventListener()
method takes at least two parameters. The first is always the event it's listening for. See here for a full list of the event keywords. The parameters after that are the functions that will be triggered, separated by a comma if there are more than one.
Passing paramaters into EventListner functions
Like the above event handlers, you need to omit the () part of the functions you place in the event listener. Otherwise, they just automatically execute when that the browser reads that part of the code which is probably not what you want. But there's a problem: How do you pass parameters to the functions you bind to events? Just wrap them in an anonymous function!
element.addEventListener('click', function(){function1(parameters...)});
Troubleshooting your Script
- Inserting
alert()
statements at key points in your script. You can use these to alert you on the status of important variables so you can follow along your code logic as it progresses. - Most browsers now have developer tools that you can use to debug your script. A DOM explorer allows you to see all your DOM objects and make direct changes to the HTML code while viewing those changes on the web page in real time. There's also a console that lets you view any errors or warnings that may have occured while running your script. You can trigger console messages for any events in your code using
console.log()
at key points in your script. Part of the developer toolkit also includes a debugger, which lets you go step by step through the JS code and view the values of any variables as well as the logic path. - Use the browser's built in debugger tool to step through your code line-by-line. This will let you see all the variables and data as they are processed by your script and help you figure out any pitfalls with your code logic.
- You can use online script evaluators which go through you code and evaluate it syntactically. A good one is www.jshint.com.
- Sometimes, it's impossible to prevent all the errors your program can have, especially when your site starts asking for user input. In this case, use a
try...catch
statement.